Machine Learning & AI for Robotics
Mini-Projects Overview
The following mini-projects were completed throughout the duration of EECS 469: Machine Learning & Artificial Intelligence for Robotics. Each explores a different aspect of robot estimation and planning (Bayesian filtering, Machine Learning, Artificial Intelligence, Localization, Motion Planning, etc), and focuses predominantly on how these subjects pertain to mobile robots. All code was written completely from scratch, to ensure a deep understanding of the material. See below for an overview of some of the mini-projects!
Skills Involved
- Bayesian statistics and Markov Processes
- Programming in Python
- Algorithm and data structure development
- Working with real robot data
- Dealing with noisy measurements and missing/asynchronous data
- Machine Learning development, setup, training, and implementation
- Algorithm performance evaluation and validation
- Framing problems appropriately for analysis with different Machine Learning & AI techniques
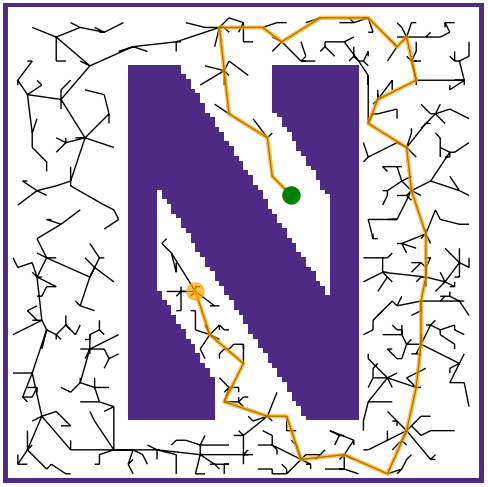
RRT Search Algorithm
A Rapidly-exploring Random Tree (RRT) is a simple and computationally-efficient search algorithm. Developed at Iowa State (my undergraduate Alma mater), this algorithm commonly augments a more hefty primary search method (e.g. A*).
The adjacent graphic illustrates my RRT navigating Northwestern's 'N' logo. The initial and goal positions are shrouded by the N, which can be computationally expensive for some algorithms to handle. An RRT can generate a rough path quickly and later smooth it for traversal, or use it to guide a slower, but highly-optimized primary algorithm.
Get the code here.
A* Search Algorithm
The A* algorithm is a popular AI search algorithm for robot motion planning. The project focused on developing an "online" variant, which could only observe adjacent cells, to allow the simulated robot to plan as it moved. The challenge was to formulate an optimized distance formula and heuristic algorithm, two of the biggest design considerations with A*, to navigate a 2D field with obstacles. The graphic shows the results of the algorithm circumnavigating an arbitrary barrier setup with ease.
Once A* had planned the path, an IK (Inverse Kinematic) motion controller was implemented to drive an imaginary puck-style robot along the route. Many challenges had to be overcome, including tuning the controller to account for dynamic considerations (e.g. max velocity and acceleration constraints).
Get the code here.
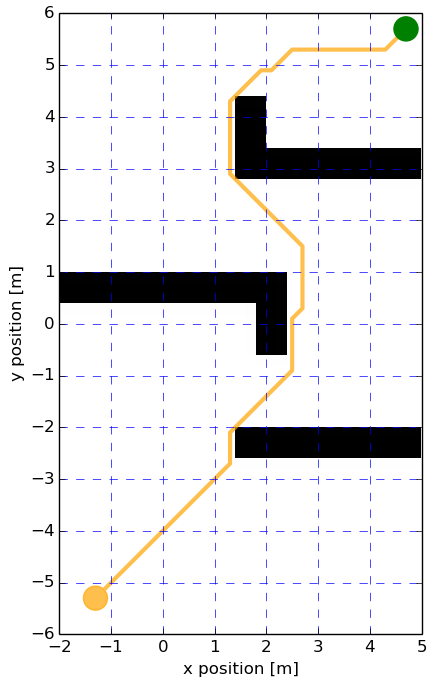
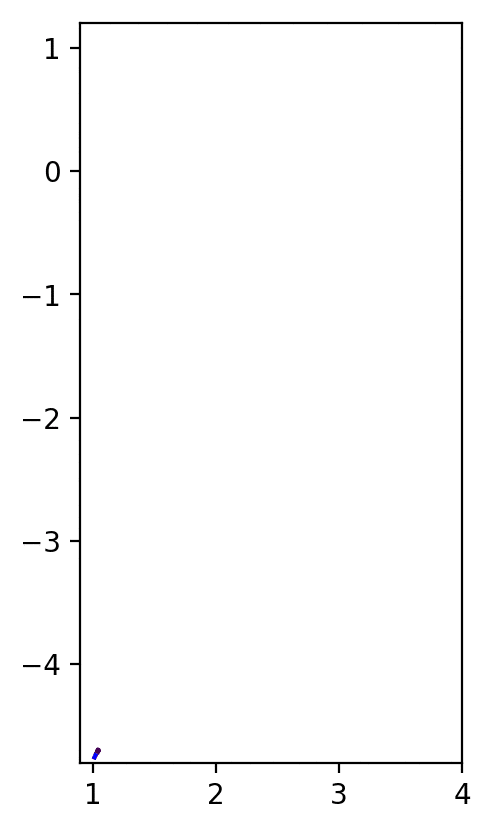
Monte Carlo Localization
Monte Carlo Localization (MCL) is a powerful localization algorithm. At the core of MCL is a particle filter, a non-parametric approximation of a Bayesian filter (e.g. Kalman). Particle filters represent the robot's posterior by particles, or individual possible states of the robot.
The accompanying animation shows the particle filter I implemented (blue line) correctly tracking the ground truth path (green line). For reference, the purely dead-reckoned path is shown in red. Looking very closely at the forefront of the blue path, you can make out the particle cloud (black arrows), although they're hard to distinguish due to their density - there are 500 in close quarters. If you observe closely, you'll see the particles splay out each step, and those towards the edge of the cloud eventually disappear after the resampling step.
Localization techniques such as this are at the core of SLAM and autonomous navigation technology, and implementing one from scratch was a great learning experience!
Check out the implementation here.
Neural Networks & LWLR
For this project, two Machine Learning algorithms were implemented and explored. Neural Networks and Locally Weighted Linear Regression (LWLR) were both tested on a data set from a prior mini-project. The goal was to learn and eliminate any hidden systematic error in a robot's dead-reckoned path. As can be seen in the accompanying figure, both algorithms were able to outperform pure dead reckoning.
Much was learned by applying two very different algorithms to the same data, and the primary purpose of the exercise was to compare the similarities and differences between them. Each group presented their findings, and each student gained a first glimpse into the speed, effectiveness, ease of use, and other important factors of many common Machine Learning algorithms.
Get the code here.
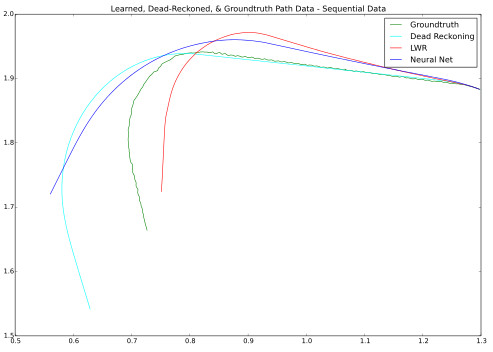
Learn More
The course is taught by Professor Brenna Argall, director of the Assistive & Rehabilitation Robotics Laboratory (argallab) at the Shirley Ryan AbilityLab (formerly Rehabilitation Institue of Chicago). Her research explores the union of human/robot interfacing, Machine Learning, and adaptable levels of autonomy. Check out the novel work being done by Dr. Argall and her team at https://www.sralab.org/labs/assistive-rehabilitation-robotics-laboratory.